Control Flow in JavaScript: If, Else, and Switch Statements for Game Development
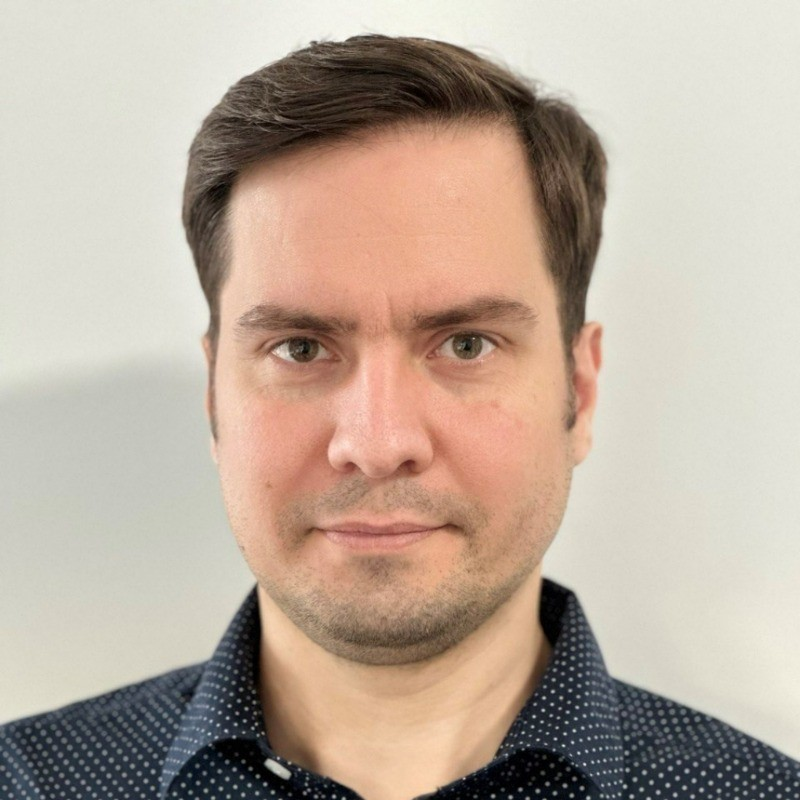
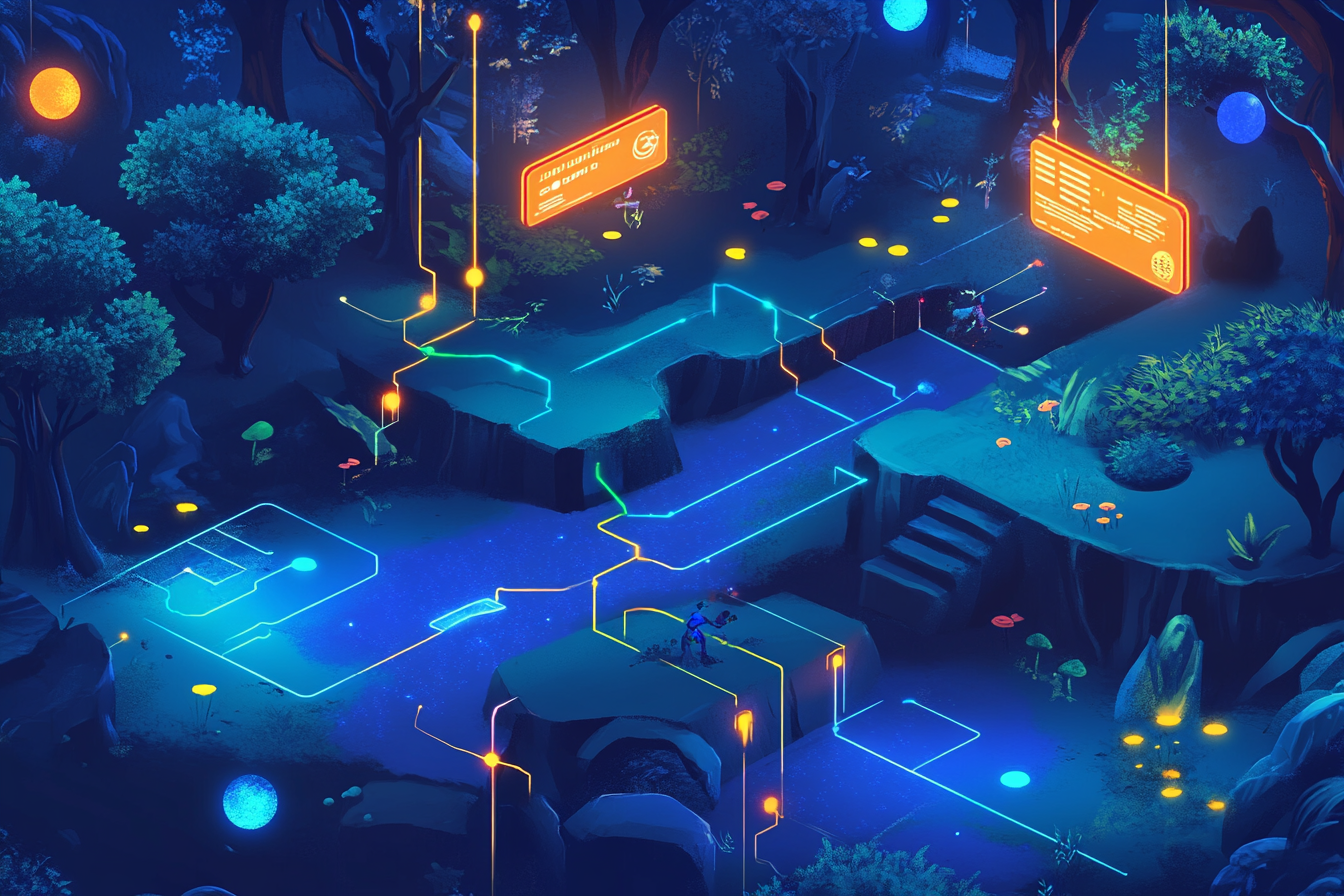
Control flow structures like if, else, and switch statements are crucial for managing decision-making in JavaScript, especially in game development. Learn how to use them to create dynamic and interactive gameplay.
Summary
In game development, control flow structures are indispensable. They allow you to guide your game’s logic, make decisions, and respond to player actions. In this article, we’ll explore how to use if
, else
, and switch
statements to implement control flow effectively in your JavaScript games.
What Is Control Flow?
Control flow refers to the order in which individual statements, instructions, or functions are executed or evaluated. In games, this might mean deciding what happens when a player takes an action, determining if the player wins or loses, or triggering different events based on the state of the game.
Using if
Statements in Games
The if
statement is used to execute a block of code only if a specified condition is true
.
Example: Checking Player Health
let playerHealth = 20;
if (playerHealth > 0) {
console.log("Player is still alive!");
}
In this case, the message will only display if the player’s health is above 0.
Adding else
for Alternate Actions
The else
statement specifies a block of code to be executed if the condition is false
.
Example: Determining Game Over State
if (playerHealth > 0) {
console.log("Player is still alive!");
} else {
console.log("Game Over!");
}
This ensures the game reacts appropriately whether the player survives or not.
Combining Conditions with else if
The else if
statement allows you to check multiple conditions.
Example: Checking Game States
let score = 100;
if (score >= 200) {
console.log("You reached the high score!");
} else if (score >= 100) {
console.log("Keep going! You're halfway there!");
} else {
console.log("Try harder!");
}
Here, the game responds differently depending on the player’s score.
Using Logical Operators in Conditions
Logical operators allow you to combine multiple conditions.
Example: Unlocking a Special Level
let hasKey = true;
let reachedGate = true;
if (hasKey && reachedGate) {
console.log("Level unlocked!");
} else {
console.log("Find the key first!");
}
Logical operators like &&
(AND) and ||
(OR) make conditions more versatile.
Using switch
Statements in Games
The switch
statement is ideal when you have many possible values for a single variable and want to execute different blocks of code for each value.
Example: Character Selection
let character = "mage";
switch (character) {
case "warrior":
console.log("You selected the Warrior! Prepare for battle.");
break;
case "mage":
console.log("You selected the Mage! Master the arcane.");
break;
case "archer":
console.log("You selected the Archer! Aim true.");
break;
default:
console.log("Please select a valid character.");
}
This provides a clean and readable way to handle multiple options.
Practical Examples in Game Development
Example 1: Managing Player Lives
let lives = 3;
if (lives > 0) {
console.log("You have " + lives + " lives remaining.");
} else {
console.log("Game Over!");
}
Example 2: Determining Game Difficulty
let difficulty = "hard";
switch (difficulty) {
case "easy":
console.log("Enemies move slower.");
break;
case "medium":
console.log("Enemies have normal speed.");
break;
case "hard":
console.log("Enemies are faster and stronger!");
break;
default:
console.log("Invalid difficulty level.");
}
Example 3: Checking for Power-Ups
let hasShield = true;
let hasDoublePoints = false;
if (hasShield || hasDoublePoints) {
console.log("You have a power-up active!");
} else {
console.log("No power-ups available.");
}
Conclusion
Control flow structures like if
, else
, and switch
statements are essential for creating dynamic and responsive game mechanics. They allow you to handle player decisions, manage game states, and implement complex logic with ease.
Key Takeaways:
- Use
if
statements for basic conditions. - Combine
if
,else
, andelse if
for multi-condition logic. - Use
switch
statements for cleaner multi-option scenarios. - Apply logical operators for more advanced conditions.
Challenge: Write a program where a player collects items and triggers different outcomes based on the items they collect. Use if
, else
, and switch
statements to implement the logic.
Happy Game Development!
Comments