Defining and Invoking Functions in JavaScript for Game Development
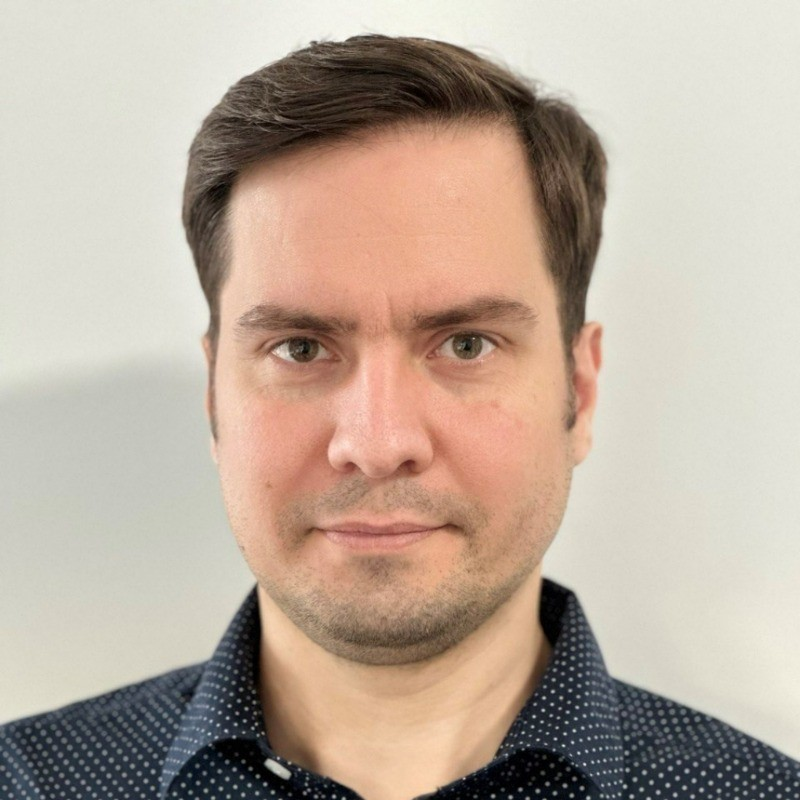
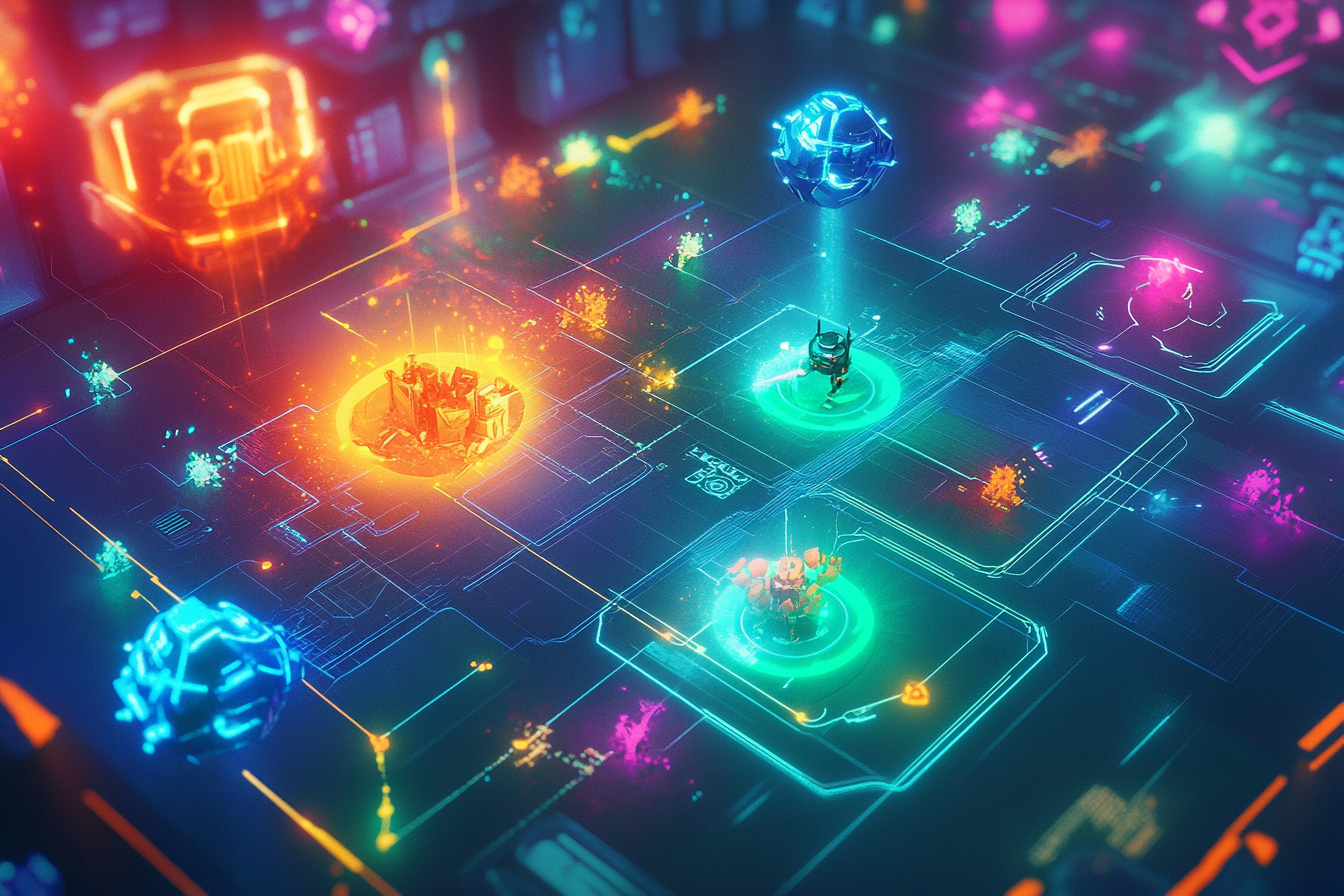
Functions are the backbone of reusable and organized code in JavaScript, especially in game development. Learn how to define and invoke functions to build dynamic and efficient game mechanics.
Summary
Functions are one of the most fundamental concepts in JavaScript and are critical in game development. They allow you to encapsulate reusable blocks of code, simplify complex logic, and create dynamic game mechanics like movement, scoring, and enemy behavior. In this article, we’ll explore how to define and invoke functions to make your games more organized and interactive.
What Are Functions?
A function is a block of reusable code designed to perform a specific task. Functions make your code modular, readable, and easy to maintain. In game development, you can use functions for tasks like:
- Moving a character
- Calculating scores
- Spawning enemies
- Checking game conditions
Defining Functions in JavaScript
You can define a function in JavaScript using the function
keyword or as an arrow function (ES6+).
Basic Syntax
function functionName(parameters) {
// Code to execute
}
Example: A Simple Scoring Function
function calculateScore(baseScore, bonusPoints) {
return baseScore + bonusPoints;
}
Invoking Functions
To use a function, you need to “call” or “invoke” it by using its name followed by parentheses. If the function has parameters, you pass the required arguments within the parentheses.
Example: Calling the Scoring Function
let score = calculateScore(100, 50);
console.log("Total Score: " + score);
Function Parameters and Arguments
Functions can accept inputs (parameters) and return outputs (values). This makes them flexible and powerful.
Example: Moving a Player
function movePlayer(x, y) {
console.log(`Player moved to position (${x}, ${y})`);
}
movePlayer(10, 20);
Default Parameters
You can assign default values to function parameters in case no arguments are provided during invocation.
Example: Attacking an Enemy
function attackEnemy(damage = 10) {
console.log(`Enemy took ${damage} damage!`);
}
attackEnemy(); // Default damage
attackEnemy(20); // Custom damage
Return Values
Functions can return values to the caller, making them reusable for calculations and decisions.
Example: Calculating Health
function calculateHealth(currentHealth, damage) {
return currentHealth - damage;
}
let health = calculateHealth(100, 25);
console.log("Remaining Health: " + health);
Function Expressions
Functions can also be defined as expressions and stored in variables.
Example: Healing a Player
const healPlayer = function(health, healingAmount) {
return health + healingAmount;
};
console.log(healPlayer(50, 20)); // 70
Arrow Functions
Arrow functions provide a shorter syntax for writing functions.
Example: Generating a Random Enemy
const generateEnemy = () => {
console.log("Enemy spawned!");
};
generateEnemy();
Practical Examples for Game Development
Example 1: Player Movement Function
function moveCharacter(direction, steps) {
console.log(`Player moved ${steps} steps to the ${direction}`);
}
moveCharacter("left", 3);
moveCharacter("right", 5);
Example 2: Enemy Attack Function
function enemyAttack(damage) {
console.log(`Enemy deals ${damage} damage!`);
}
enemyAttack(15);
enemyAttack(20);
Example 3: Game Timer Function
function startTimer(seconds) {
let timer = seconds;
const interval = setInterval(() => {
console.log(`Time remaining: ${timer} seconds`);
timer--;
if (timer < 0) {
clearInterval(interval);
console.log("Game Over!");
}
}, 1000);
}
startTimer(10);
Conclusion
Functions are the backbone of reusable, modular code in JavaScript, especially for game development. They simplify complex logic, enhance code readability, and make your games more dynamic and interactive.
Key Takeaways:
- Functions encapsulate reusable blocks of code.
- Use parameters and return values to make functions flexible.
- Arrow functions offer a concise way to write functions.
- Organize your game logic into specific functions for better maintainability.
Challenge: Write a program that uses functions to handle player actions like moving, attacking, and collecting items. Implement reusable logic to streamline gameplay.
Happy Game Development!
Comments