Introduction to JavaScript Variables and Data Types
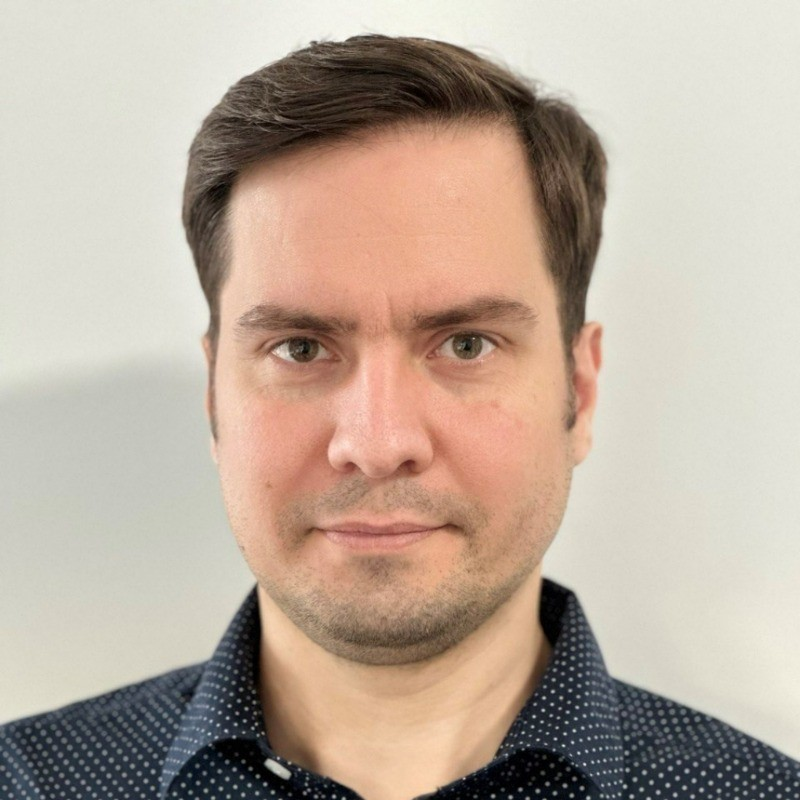
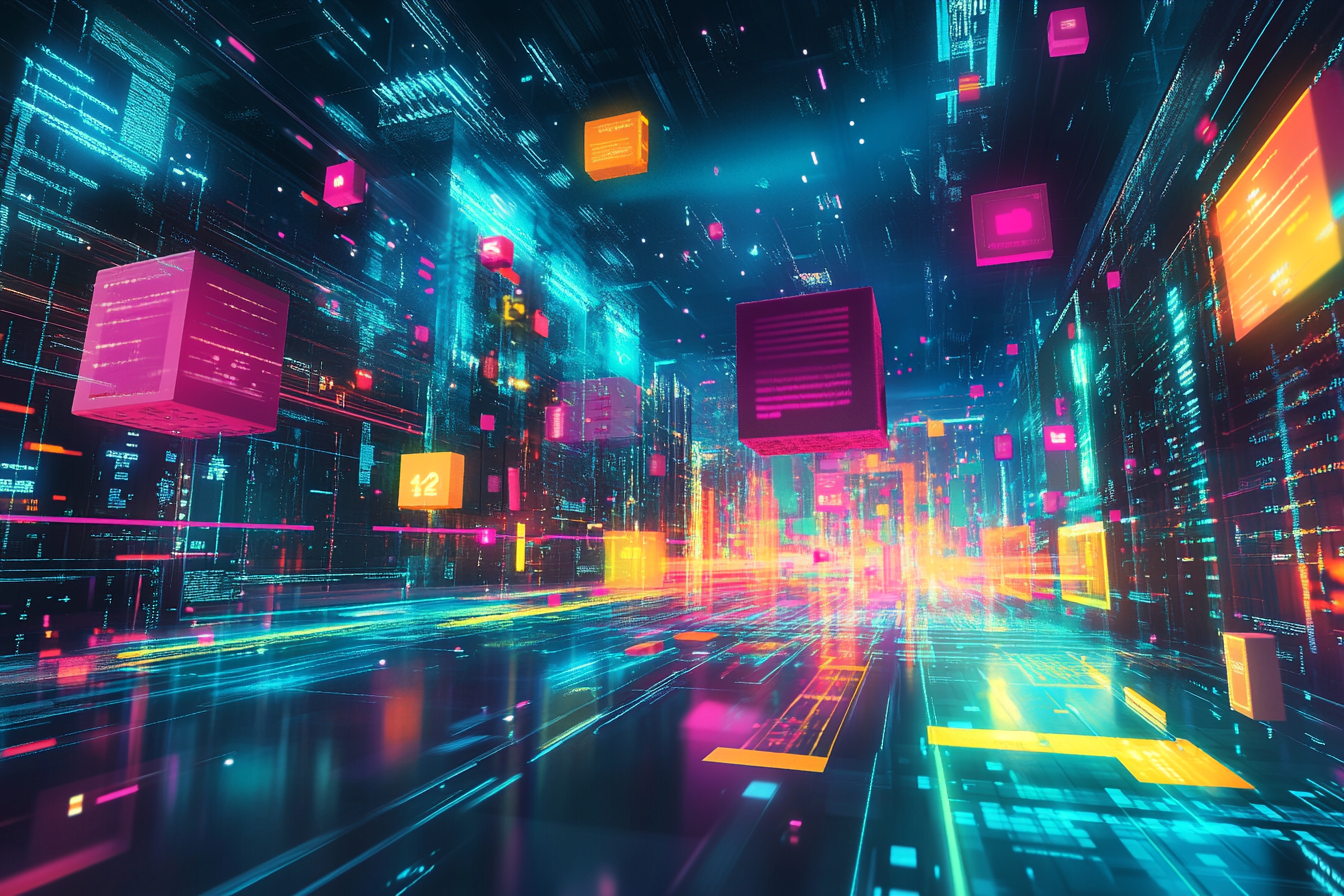
Understanding variables and data types is essential for mastering JavaScript, especially for game development. In this article, we'll explore the fundamental concepts and provide examples to kickstart your learning.
Summary
JavaScript variables and data types are the foundation of any program you create. They allow you to store and manipulate information, whether it’s a player’s score, game settings, or character details. Let’s dive into the basics and see how they can be used effectively.
What Are Variables?
Variables in JavaScript are containers for storing data. Think of them as labeled boxes where you keep information your program needs to work with.
Declaring Variables in JavaScript
JavaScript allows you to declare variables using three keywords: var
, let
, and const
.
Using var
The var
keyword is function-scoped and was the original way to declare variables in JavaScript.
var playerName = "Alex";
var score = 0;
Using let
Introduced in ES6, let
is block-scoped and is recommended for most cases.
let lives = 3;
let isGameOver = false;
Using const
Also introduced in ES6, const
is used for declaring variables that won’t change.
const MAX_LEVEL = 10;
const GRAVITY = 9.8;
Note: While the variable identifier cannot be reassigned, the contents of objects or arrays declared with
const
can still be modified.
Understanding Data Types
JavaScript variables can hold many types of data, broadly categorized into primitive and reference types.
Primitive Data Types
-
Number
Represents numeric values, including integers and floating-point numbers.
let score = 100; let temperature = 36.5;
-
String
Represents text.
let playerName = "Alex";
-
Boolean
Represents logical values:
true
orfalse
.let isGameOver = false;
-
Undefined
A variable declared but not assigned a value.
let x; console.log(x); // undefined
-
Null
Represents the intentional absence of a value.
let selectedItem = null;
-
Symbol (ES6)
A unique and immutable data type.
let uniqueId = Symbol('id');
-
BigInt (ES2020)
For integers beyond the safe integer range.
let largeNumber = 12345678901234567890n;
Reference Data Types
-
Object
A collection of key-value pairs.
let player = { name: "Alex", score: 0, lives: 3 };
-
Array
Ordered list of values.
let inventory = ["sword", "shield", "potion"];
Type Conversion
JavaScript is dynamically typed, meaning variables can change their type during runtime. This can happen implicitly or explicitly.
Implicit Conversion
JavaScript converts types automatically in certain operations.
let result = "5" + 2; // "52"
Explicit Conversion
You can manually convert types using functions like Number()
, String()
, or Boolean()
.
let str = "123";
let num = Number(str); // 123
Practical Examples for Game Development
Example 1: Storing Player Information
let playerName = "Alex";
let score = 0;
let lives = 3;
let isGameOver = false;
Example 2: Updating Game State
function updateScore(points) {
score += points;
}
function loseLife() {
lives -= 1;
if (lives === 0) {
isGameOver = true;
}
}
Example 3: Using Constants
const MAX_LIVES = 5;
const GRAVITY = 9.81;
Example 4: Working with Objects
let player = {
name: "Alex",
score: 0,
lives: 3,
inventory: ["sword", "shield"],
};
function addItem(item) {
player.inventory.push(item);
}
Conclusion
Variables and data types are the cornerstone of JavaScript programming. Understanding them is crucial for writing efficient and effective code, especially in game development.
Key Takeaways:
- Use
let
andconst
for better scoping and immutability. - Understand primitive and reference data types.
- Be mindful of type conversion to avoid unexpected behavior.
Challenge: Write a small program that tracks a player’s score and inventory. Include functions to add points and items, and display the updated data in the console.
Happy Coding!
Comments