Understanding Operators and Expressions in JavaScript for Game Development
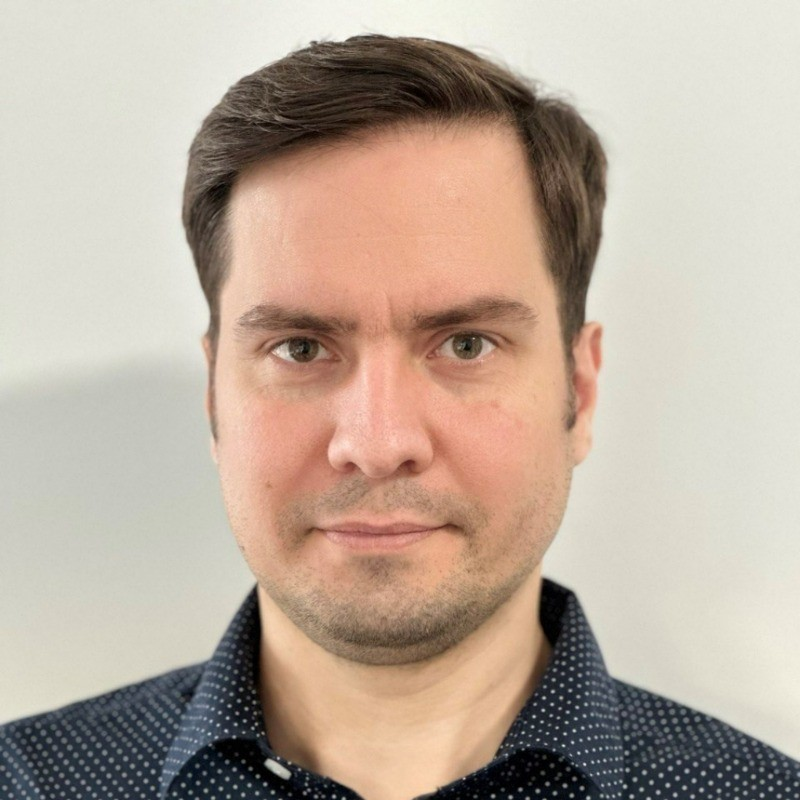
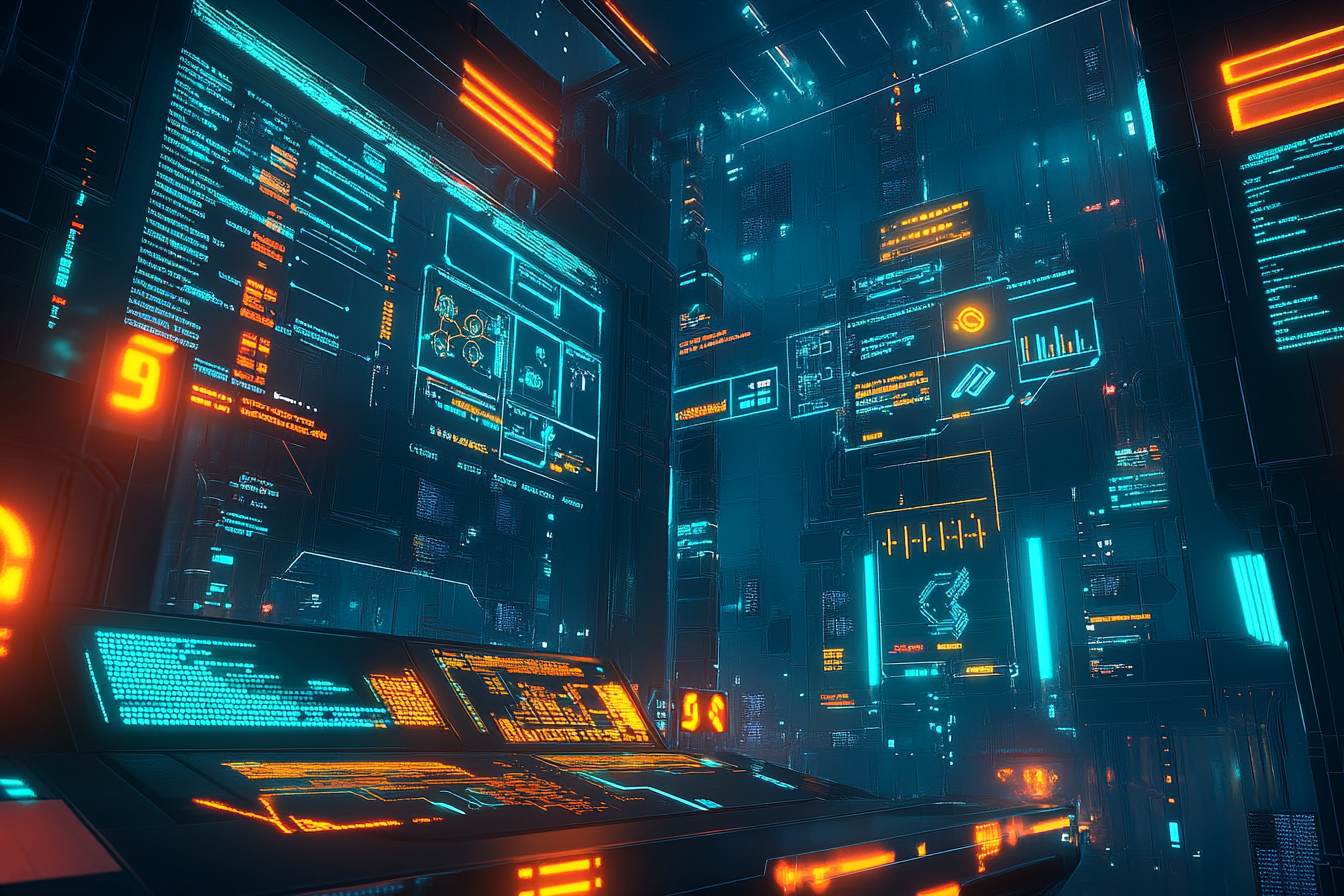
Operators and expressions are key tools for creating interactive game mechanics in JavaScript. This article explores how to use them effectively in game development, with examples that bring your games to life.
Summary
In JavaScript game development, operators and expressions are essential for building game mechanics, controlling the flow of logic, and creating dynamic interactions. They help power everything from calculating scores to determining collision outcomes. Let’s break down these concepts and see how they directly relate to creating games.
What Are Expressions?
In the context of game development, an expression is any piece of code that calculates or determines a value, often used to control the game logic.
Examples of Expressions in Games:
- Calculating a player’s score:
let score = baseScore + bonusPoints;
- Checking if a player has enough health to survive:
let isAlive = health > 0;
- Determining the next position of a character:
let newPosition = currentPosition + speed * deltaTime;
What Are Operators?
Operators allow you to perform operations on values and variables, enabling game mechanics like movement, scoring, and decision-making.
1. Arithmetic Operators in Games
Arithmetic operators are used for calculations, such as movement, damage, or scoring.
Operator | Description | Example | Use Case |
---|---|---|---|
+ |
Addition | score + bonusPoints |
Calculating final scores |
- |
Subtraction | playerHealth - damageTaken |
Reducing health after an attack |
* |
Multiplication | speed * deltaTime |
Calculating distance traveled |
/ |
Division | totalPoints / numberOfPlayers |
Averaging scores |
% |
Modulus | time % 60 |
Formatting time in minutes/seconds |
2. Assignment Operators in Games
Assignment operators help update values dynamically during gameplay.
Operator | Description | Example | Use Case |
---|---|---|---|
= |
Assign | score = 100; |
Initializing a score |
+= |
Add and assign | score += 10; |
Awarding bonus points |
-= |
Subtract and assign | lives -= 1; |
Losing a life after a failure |
*= |
Multiply and assign | speed *= 2; |
Doubling speed during a power-up |
/= |
Divide and assign | damage /= 2; |
Halving damage with a shield |
3. Comparison Operators in Games
These operators are crucial for decision-making and logic branching in games.
Operator | Description | Example | Use Case |
---|---|---|---|
== |
Equal to | lives == 0 |
Checking if the player is out of lives |
!= |
Not equal to | score != highScore |
Determining if a new high score was achieved |
< |
Less than | enemyHealth < 10 |
Triggering a “low health” state |
> |
Greater than | score > targetScore |
Checking if the player has won |
<= |
Less than or equal to | timeLeft <= 0 |
Ending the game when time runs out |
>= |
Greater than or equal to | level >= maxLevel |
Checking if the game is complete |
4. Logical Operators in Games
Combine conditions to create more complex game logic.
Operator | Description | Example | Use Case |
---|---|---|---|
&& |
Logical AND | isAlive && hasAmmo |
Allowing shooting only if alive and armed |
|| |
Logical OR | isPlayer || isNPC |
Allowing interaction with players or NPCs |
! |
Logical NOT | !isPaused |
Resuming game when not paused |
5. Ternary Operator in Games
The ternary operator is great for concise conditional logic.
let gameState = lives > 0 ? "Playing" : "Game Over";
6. String Operators in Games
Used for creating dynamic messages or labels.
let displayMessage = "Level " + currentLevel + " Complete!";
Operator Precedence in Game Logic
When using multiple operators in a single expression, JavaScript evaluates them based on operator precedence.
Example:
let total = baseScore + bonusPoints * multiplier; // Multiplication occurs first.
Use parentheses to ensure clarity:
let total = (baseScore + bonusPoints) * multiplier;
Practical Examples for Game Development
Example 1: Calculating Final Score
let baseScore = 100;
let timeBonus = 50;
let totalScore = baseScore + timeBonus;
console.log("Your total score is: " + totalScore);
Example 2: Controlling Player Movement
let position = 0;
let speed = 5;
let deltaTime = 0.016; // Time since the last frame
position += speed * deltaTime;
console.log("Player's new position: " + position);
Example 3: Checking Win Conditions
let score = 150;
let targetScore = 200;
if (score >= targetScore) {
console.log("You win!");
} else {
console.log("Keep going!");
}
Example 4: Implementing Power-Ups
let speed = 5;
let isPoweredUp = true;
speed = isPoweredUp ? speed * 2 : speed;
console.log("Player's speed: " + speed);
Conclusion
Understanding operators and expressions in JavaScript is a must for game developers. They power critical game mechanics like scoring, movement, and decision-making, allowing you to create engaging and dynamic gameplay.
Key Takeaways:
- Use arithmetic operators for calculations like movement and scoring.
- Apply comparison and logical operators to control game logic.
- Leverage operator precedence to write clear and efficient code.
Challenge: Write a game mechanic where the player collects items, and the score increases based on the rarity of the item. Use arithmetic and comparison operators to implement and display the logic.
Happy Game Development!
Comments