Loops in JavaScript: For, While, and Do-While for Game Development
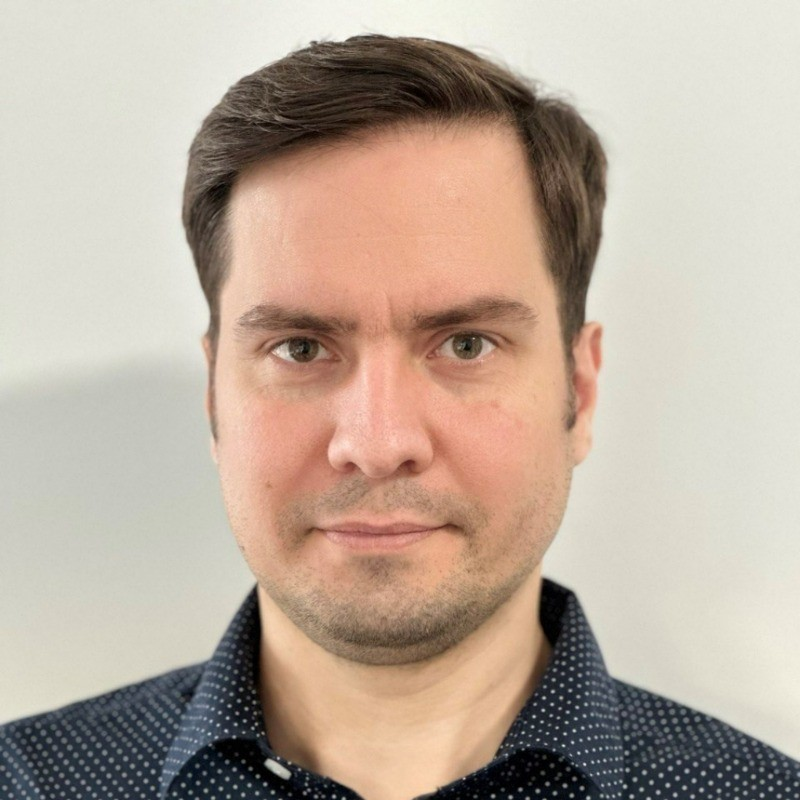
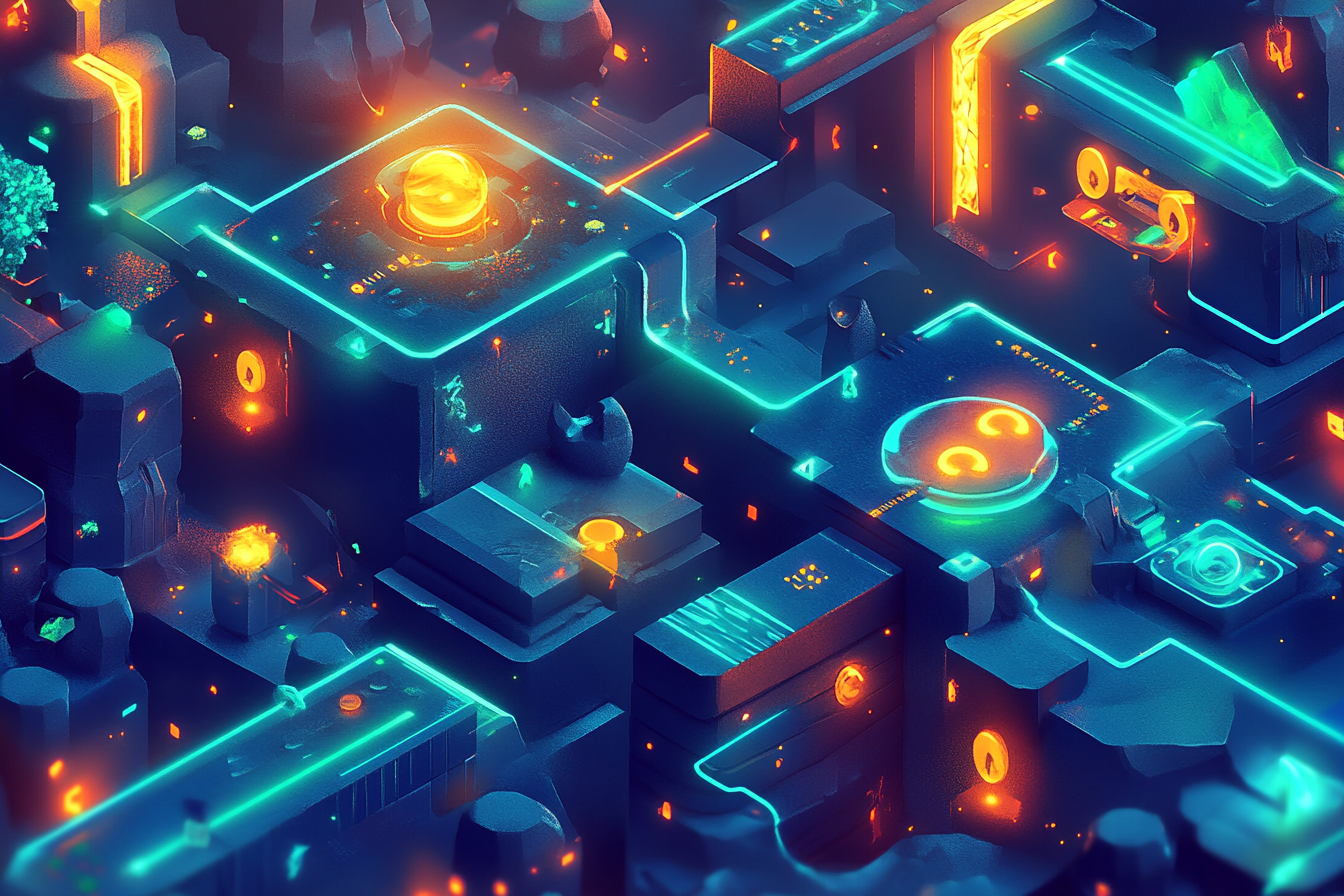
Loops are essential tools in JavaScript game development, enabling you to repeat actions like updating the game state, checking conditions, and iterating through data. Learn how to use for, while, and do-while loops effectively in your games.
Summary
In JavaScript game development, loops are powerful tools that allow you to repeat actions, iterate over data, and manage game states efficiently. Whether you’re creating a dynamic enemy spawn system, iterating over game objects, or building a scoring system, loops are your go-to mechanism. In this article, we’ll explore the three main types of loops in JavaScript: for
, while
, and do-while
.
What Are Loops?
A loop is a control structure that allows you to execute a block of code repeatedly, either for a fixed number of times or until a condition is met. In games, loops are commonly used to update game states, process game objects, or handle repetitive tasks.
The for
Loop
The for
loop is ideal when you know the exact number of iterations in advance. It is often used to iterate through arrays or execute a block of code a specific number of times.
Syntax
for (initialization; condition; increment) {
// Code to execute
}
Example: Iterating Over an Inventory
let inventory = ["sword", "shield", "potion"];
for (let i = 0; i < inventory.length; i++) {
console.log("You have: " + inventory[i]);
}
Example: Spawning Multiple Enemies
for (let i = 1; i <= 5; i++) {
console.log("Spawning enemy #" + i);
}
The while
Loop
The while
loop is used when the number of iterations is unknown, but a condition needs to be checked before each iteration.
Syntax
while (condition) {
// Code to execute
}
Example: Decreasing Player Health
let health = 100;
while (health > 0) {
console.log("Player health: " + health);
health -= 10;
}
console.log("Game Over!");
Example: Respawning Until Conditions Are Met
let enemiesRemaining = 3;
while (enemiesRemaining > 0) {
console.log("Enemy respawned!");
enemiesRemaining--;
}
The do-while
Loop
The do-while
loop is similar to the while
loop but ensures that the block of code is executed at least once before the condition is checked.
Syntax
do {
// Code to execute
} while (condition);
Example: Rolling a Dice Until a Six is Rolled
let diceRoll;
do {
diceRoll = Math.floor(Math.random() * 6) + 1;
console.log("Rolled: " + diceRoll);
} while (diceRoll !== 6);
console.log("You rolled a six!");
Example: Player Actions Menu
let action;
do {
action = prompt("Choose an action: attack, heal, or run:");
console.log("Player chose: " + action);
} while (action !== "run");
console.log("Player escaped!");
Choosing the Right Loop for Your Game
- Use a
for
loop when you know the exact number of iterations (e.g., iterating over a game level’s objects). - Use a
while
loop when you need to repeat an action until a condition is false (e.g., reducing enemy health). - Use a
do-while
loop when you need the code to execute at least once before checking a condition (e.g., player input).
Practical Examples in Game Development
Example 1: Iterating Over Game Levels
let levels = ["Forest", "Cave", "Castle"];
for (let i = 0; i < levels.length; i++) {
console.log("Entering level: " + levels[i]);
}
Example 2: Countdown Timer for a Game Start
let countdown = 5;
while (countdown > 0) {
console.log("Game starts in: " + countdown + " seconds");
countdown--;
}
console.log("Go!");
Example 3: Implementing a Treasure Hunt
let treasureFound = false;
do {
console.log("Searching for treasure...");
treasureFound = Math.random() > 0.8; // 20% chance to find treasure
} while (!treasureFound);
console.log("Treasure found!");
Conclusion
Loops are an essential part of JavaScript game development. They simplify repetitive tasks, allow you to manage game logic efficiently, and create dynamic gameplay experiences. Mastering loops will make your games more robust and interactive.
Key Takeaways:
- Use
for
loops for fixed iterations like iterating over game objects. - Use
while
loops for conditions that need to be checked before each iteration. - Use
do-while
loops when the code block must execute at least once.
Challenge: Create a loop that simulates a player’s journey through a dungeon. The loop should iterate through rooms and display messages about the player’s progress until they reach the treasure.
Happy Game Development!
Comments